Objective:
An android app where i can login using Facebook and connect the login information with Firebase Authentication.
Step 1: Create and Run a basic React Native app.
(You can just follow this for Step 1: https://facebook.github.io/react-native/docs/getting-started.html#content )
Navigate to any folder where you want to create the project and use react native init.

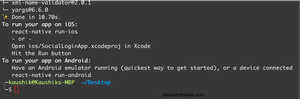
Yarn ye! No world didnt changed for me… yet!!
For this tutorial we will focus on Android, but iOS is kind of similar.
cd to project folder and
╰─$ react-native run-android
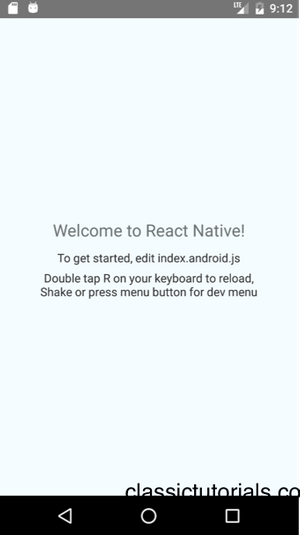
Step 2: Creating a less ugly screen
index.android.js
<View style={styles.container}> <View style={styles.top}> <Text style={styles.textheader}> Social Login </Text> </View> <View style={styles.bottom}> <Text> Button will come here </Text> </View> </View> //Styling: const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#1C2833', alignItems: 'center', flexDirection:'column', justifyContent: 'center', }, top:{ flex:1, justifyContent: 'center', }, bottom:{ flex:1, }, textheader:{ color:'white', fontSize: 50 } });
Step 3: Facebook SDK
Execute following on your project folder, we will use official fb repo:
react-native install react-native-fbsdk
react-native link react-native-fbsdkShould take sometime based on n/w speed.
Assume you have Android Studio, else highly suggest you install it now.
Open the Android folder as android project in Android Studio. I will be using Android studio 2.2
In MainApplication.java add an instance of CallbackManager with getter; CallbackManager manages the callback to FB SDK.
private static CallbackManager mCallbackManager = CallbackManager.Factory.create(); protected static CallbackManager getCallbackManager() { return mCallbackManager; }than under getPackages pass the newly created callback manager to FBSDK package.
@Override protected List<ReactPackage> getPackages() { return Arrays.<ReactPackage>asList( new MainReactPackage(), new FirestackPackage(), new FBSDKPackage(mCallbackManager) ); } };In MainActivity.java make sure you already have the following method. The SDK react link should have created this.
import android.content.Intent;
@Override public void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); MainApplication.getCallbackManager().onActivityResult(requestCode, resultCode, data); }
Now we will create an FB app which will handle our login flow.
Navigate to https://developers.facebook.com/quickstarts/?platform=android and follow the onscreen instruction.
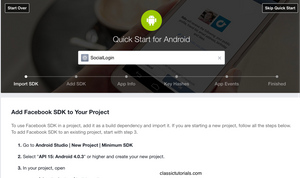
You can either do the remaining few steps from FB or just read below instructions. Both are kind of same.
in android/build.gradle add
mavenCentral()So it should look like this.
allprojects { repositories { mavenLocal() jcenter() maven { // All of React Native (JS, Obj-C sources, Android binaries) is installed from npm url "$rootDir/../node_modules/react-native/android" } mavenCentral() } }in android/app/build.gradle add the following for Facebook SDK
compile 'com.facebook.android:facebook-android-sdk:[4,5)'
So it should look like,
dependencies { compile project(':react-native-firestack') compile project(':react-native-fbsdk') compile fileTree(dir: "libs", include: ["*.jar"]) compile "com.android.support:appcompat-v7:23.0.1" compile "com.facebook.react:react-native:+" // From node_modules compile 'com.facebook.android:facebook-android-sdk:[4,5)' }Now sync your Gradle files on Android Studio.
Now open String.xml and add facebook_app_id. You can find the id from facebook page.
app/res/values/String.xml
<resources> <string name="app_name">SocialLoginApp</string> <string name="facebook_app_id">%%%%6071338448%%</string> </resources>Open AndroidManifest.xml and add a meta-data element to the application element:
<meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/>Should look something like this.
</activity> <activity android:name="com.facebook.react.devsupport.DevSettingsActivity" /> <meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/> </application>Then update the android project info on FB console.
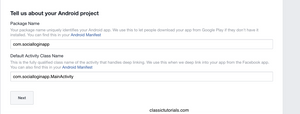
Click next, it will ask for hash key, on your terminal execute this:
keytool -exportcert -alias YOUR_RELEASE_KEY_ALIAS -keystore YOUR_RELEASE_KEY_PATH | openssl sha1 -binary | openssl base64
You can close the Android studio now. Moving to react project:
Now open index.android.js
Add the following to import FB SDK.
const FBSDK = require('react-native-fbsdk'); const { LoginButton, AccessToken } = FBSDK;
and then replace “ Button will come here ” text component section with the following code.
<LoginButton onLoginFinished={ (error, result) => { if (error) { console.log("login has error: " + result.error); } else if (result.isCancelled) { console.log("login is cancelled."); } else { AccessToken.getCurrentAccessToken().then( (data) => { console.log(data); } ) } } } onLogoutFinished={() => console.log("logout.")}/>Think the code is pretty self explanatory
Stop the React project and re run it again to load all libs etc.
Click on the button and make sure its log-in in and showing you the logout button. If thats working all set. (Alternatively check the chrome console for the console log of data)
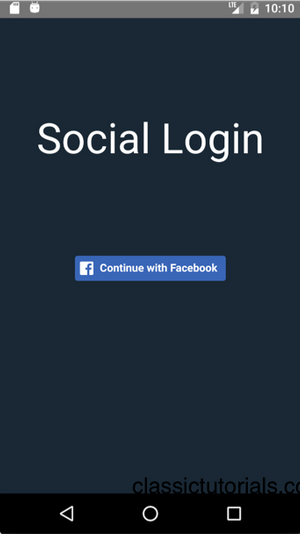
Step 4: Firebase integration
For firebase integration we will use Firestack.
Within the main react project folder run the following>
npm install react-native-firestack --savefollowed by
react-native link react-native-firestack
Now keep your android studio open while navigate to https://console.firebase.google.com/
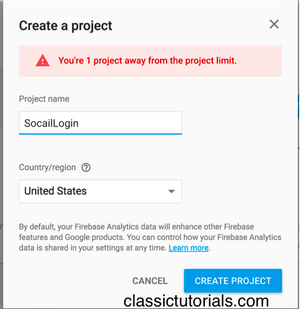
Click ADD APP and download the google-service.json
Now in Firebase under Authentication enable Facebook. All FB related info like app id and secret key you will get it from FB console from the app previously created.
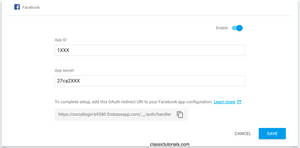
Add the JSON file under the main project.
Under React Project/android/app
You can see it on android project.
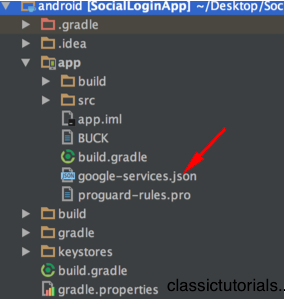
Now under android/build.gradle
under dependency add this.
classpath 'com.google.gms:google-services:3.0.0'and under android/app/build.gradle
add the following at the extreme end of the file.
apply plugin: 'com.google.gms.google-services'Try a project clean on android studio (Build>Project Clean)to make sure everything is in right order.
Cool done with Android Studio. Moving to React project now.
add the import;
import Firestack from 'react-native-firestack';
and add the initializations just after that:
const firestack = new Firestack();
Now where we received the access token from facebook we will pass the access token to Firebase.
So under
AccessToken.getCurrentAccessToken().then( (data) => {
add the following:
firestack.auth.signInWithProvider('facebook', data.accessToken, '') // facebook will need only access token. .then((user)=>{ console.log("Inside "); console.log(user); })
Thats it. Run the app. Note that Android requires the Google Play services to installed for authentication to function. So you may want to try running on a real device or configure simulator for Google Play service
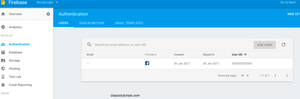
For your reference here is my final index.android.js file.
/** * Sample React Native App * https://github.com/facebook/react-native * @flow */ import React, { Component } from 'react'; import { AppRegistry, StyleSheet, Text, View } from 'react-native'; const FBSDK = require('react-native-fbsdk'); const { LoginButton, AccessToken } = FBSDK; import Firestack from 'react-native-firestack'; const firestack = new Firestack(); export default class SocialLoginApp extends Component { render() { return ( <View style={styles.container}> <View style={styles.top}> <Text style={styles.textheader}> Social Login </Text> </View> <View style={styles.bottom}> <LoginButton onLoginFinished={ (error, result) => { if (error) { console.log("login has error: " + result.error); } else if (result.isCancelled) { console.log("login is cancelled."); } else { AccessToken.getCurrentAccessToken().then( (data) => { firestack.auth.signInWithProvider('facebook', data.accessToken, '') .then((user)=>{ console.log("Inside "); console.log(user); }) } ) } } } onLogoutFinished={() => console.log("logout.")}/> </View> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#1C2833', alignItems: 'center', flexDirection:'column', justifyContent: 'center', }, top:{ flex:1, justifyContent: 'center', }, bottom:{ flex:1, }, textheader:{ color:'white', fontSize: 50 } }); AppRegistry.registerComponent('SocialLoginApp', () => SocialLoginApp);Publishing to git hub if you need?